클래스(class)라는 것을 기반으로
객체(object)를 만들고,
그러한 객체를 우선으로 생각해서
프로그래밍하는 것을 이념으로 삼고 있습니다.
객체
업무 프로그램을 만든다면
이름, 식별번호, 성적 등이 필요하며
리스트와 딕셔너리로 표현하기
# 사람 리스트 선언 people = [ {"name": "윤신종", "number": 1, "korean": 88, "math": 90}, {"name": "김이박", "number": 2, "korean": 80, "math": 95}, {"name": "박이윤", "number": 3, "korean": 90, "math": 93} ] print("이름", "총점", "평균", sep='\t') for person in people: # 점수 총합과 평균 구하기 score_sum = person["korean"] + person["math"] score_average = score_sum / 2 print(person["name"], score_sum, score_average, sep="\t")

함수로 객체 만들기
def create_person(name, number, korean, math): return { "name": name, "number": number, "korean": korean, "math": math } # 학생 리스트 선언 people = [ create_person("윤종신", 1, 99, 98), create_person("김이박", 2, 98, 22), create_person("윤이슬", 3, 100, 23), create_person("감바삭", 3, 100, 23) ] print("이름","번호","총점", sep='\t') for person in people: score_sum = person["korean"] + person["math"] print(person["name"], person["number"], score_sum, sep="\t")
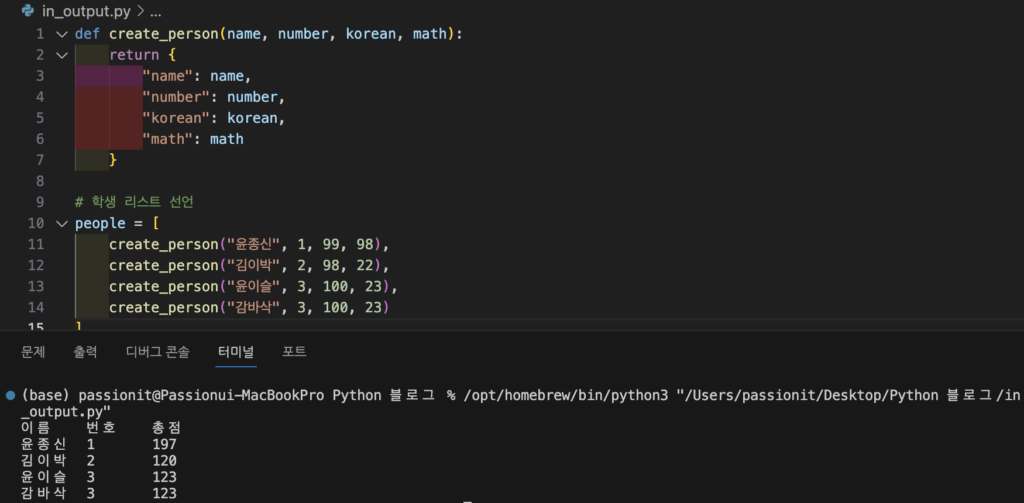
클래스 선언하기
객체를 조금 더 효율적으로 생성하기 위해 만들어진 구문입니다.
형태
class 이름: 클래스 내용
인스턴스 이름(변수 이름) = 클래스 이름() -> 생성자 함수라고 부릅니다.
클래스를 기반으로 만들어진 객체를 인스턴스(instance)라고 부릅니다.
# 선언 class Person: pass # 사람을 선언 person = Person() # 사람 리스트 선언 person = [ Person(), Person(), Person(), Person() ]
생성자
클래스 이름과 같은 함수를
생성자(constructor)라고 부릅니다.
class 클래스 이름: def __init__(self, 추가적인 매개변수): pass
클래스 내부 함수는
첫 번째 매개변수로 self를 입력해야합니다.
self는 ‘자기자신’을 나타내는 딕셔너리라고 생각하면 됩니다.
# 선언하기 class Person: def __init__(self, name, korean, math, english, science): self.name = name self.korean = korean self.math = math self.english = english self.science = science # 학생 리스트를 선언 people = [ Person("이춘백", 98, 89, 88, 95), Person("김기백", 99, 100, 50, 80), Person("김현진", 96, 56, 70, 40), Person("김이현", 98, 87, 99, 90) ] # People 인스턴스의 속성에 접근하는 방법 print(people[0].name) # 이춘백 print(people[0].korean) # 98 print(people[0].math) # 89 print(people[0].english) # 88 print(people[0].science) # 95

소멸자(__del__)
class Test: def __init__(self, name): self.name = name print("{} - 생성되었습니다.".format(self.name)) def __del__(self): print("{} - 파괴되었습니다.".format(self.name)) test = Test("A")
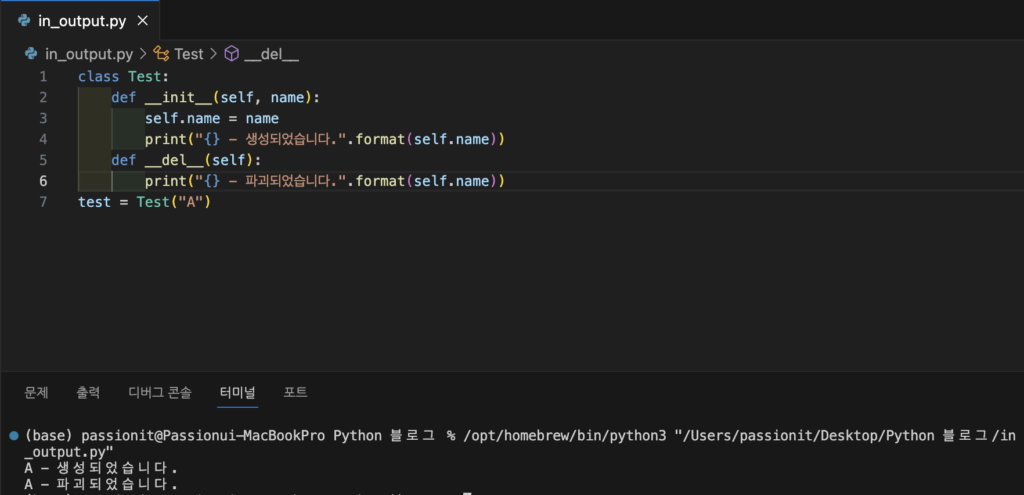
메소드
클래스가 가지고 있는 함수를
메소드(method)라고 부릅니다.
# 선언 class Person: def __init__(self, name, korean, math, english, science): self.name = name self.korean = korean self.math = math self.english = english self.science = science def get_sum(self): return self.korean + self.math + self.english + self.science def get_average(self): return self.get_sum() / 4 def to_string(self): return "{}\t{}\t{}".format(self.name, self.get_sum(), self.get_average()) students = [ Student("이춘백", 98, 89, 88, 95), Student("김기백", 99, 100, 50, 80), Student("김현진", 96, 56, 70, 40), Student("김이현", 98, 87, 99, 90) ] # 한 명씩 반복 print("이름", "총점", "평균", sep=\t) for student in students: print(student.to_string())